在Vue应用程序中,保护网站内容不被复制或滥用非常重要。添加水印是一种有效的方法,可以防止未经授权的复制或分发。本文将介绍在Vue页面中实现水印的几种方式。
使用CSS样式
最简单的方法是通过CSS样式来添加水印。您可以在Vue组件的模板文件中添加一个包含水印文本的div元素,并为其指定透明度、颜色和位置等CSS样式。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| <template> <div class="watermark"> <span>My Watermark</span> </div> <!-- rest of the component --> </template>
<style> .watermark { position: fixed; top: 0; left: 0; width: 100%; height: 100%; opacity: 0.1; z-index: -1; background-repeat: repeat; background-image: url('data:image/png;base64,iVBORw0KGg...'); /* base64 encoded image */ } .watermark span { font-family: Arial, sans-serif; font-size: 24px; color: #000000; text-align: center; display: block; margin-top: 100px; } </style>
|
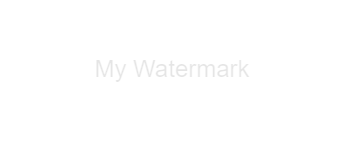
这种方法可以快速简便地添加水印,但对于图像或其他高级效果可能不够灵活。
使用HTML5 Canvas
另一种方法是使用HTML5 Canvas元素来添加自定义水印。这种方法可以实现更高级的效果,比如添加旋转或透明度等。首先在Vue组件中创建一个包含Canvas元素的div:
1 2 3 4 5 6
| <template> <div ref="watermarkContainer"> <canvas></canvas> </div> <!-- rest of the component --> </template>
|
然后在Vue的mounted钩子中使用JavaScript代码为Canvas元素添加水印:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| mounted() { const canvas = this.$refs.watermarkContainer.querySelector('canvas'); const ctx = canvas.getContext('2d');
// 设置水印样式 ctx.font = '20px'; ctx.fillStyle = '#000000'; ctx.globalAlpha = 0.5; ctx.textAlign = 'center'; ctx.rotate((Math.PI / 120));
// 添加水印文本 ctx.fillText('My Watermark', 150, 75); }
|
也可以将Canvas
转为Base64
然后设置为背景,如:
1 2
| const base64 = canvas.toDataURL("image/png"); docment.body.style = `background:url(${base64}) left top repeat`;
|
使用SVG
还有一种方法是使用SVG元素来添加自定义水印。这种方法非常易于使用和集成到现有的Vue应用程序中。首先在Vue组件中创建一个包含SVG元素的div:
1 2 3 4 5 6 7 8
| <template> <div ref="watermarkContainer"> <svg> <text x="50%" y="50%" text-anchor="middle">My Watermark</text> </svg> </div> <!-- rest of the component --> </template>
|
然后在Vue的mounted钩子中使用JavaScript代码为SVG元素添加水印:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| mounted() { const svg = this.$refs.watermarkContainer.querySelector('svg');
// 设置水印样式 svg.setAttribute('width', '100%'); svg.setAttribute('height', '100%'); svg.style.position = 'absolute'; svg.style.top = 0; svg.style.left = 0; svg.style.zIndex = '-1'; svg.style.opacity = 0.1; svg.style.pointerEvents = 'none';
const text = svg.querySelector('text'); text.setAttribute('font-size', '24'); text.setAttribute('fill', '#000000'); }
|
这三种方法都可以用于在Vue页面中添加水印。您可以根据需求和偏好选择其中之一,或者结合使用以实现更高级的效果。如果你觉得自己新写代码麻烦,没关系,现在有很多现在的开源组件
watermarkjs
watermark.js是一款用于在网页中添加水印的JavaScript库。它支持在Vue应用程序中使用,并且非常易于使用。本文将介绍如何在Vue中使用watermark.js,
安装watermark.js
可以通过npm安装watermark.js或直接引入js库:
1
| npm install watermarkjs --save
|
使用watermark.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| <template> <div> <img :src="imageUrl" /> <img :src="watermarkedImageUrl" /> </div> </template>
<script> import watermark from 'watermarkjs';
export default { name: 'WatermarkDemo', data() { return { imageUrl: 'image.jpg', watermarkedImageUrl: '' } }, mounted() { watermark([this.imageUrl]) .image(watermark.text.lowerRight('My Watermark')) .then((img) => { this.watermarkedImageUrl = img.src; }); } } </script>
|
watermark.js代码地址https://github.com/brianium/watermarkjs.git
watermark-dom
watermark-dom是一款用于在网页中添加水印的JavaScript库。它支持在Vue应用程序中使用,并且非常易于使用。
安装watermark-dom
可以通过npm安装watermark-dom或直接引入js库:
1
| npm install watermark-dom --save
|
使用watermark-dom
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| <template> <div> <img :src="imageUrl" /> </div> </template>
<script> import watermark from 'watermark-dom';
export default { name: 'WatermarkDemo', data() { return { imageUrl: 'image.jpg' } }, mounted() { watermark.init({ watermark_txt: "admin" }); } } </script>
|
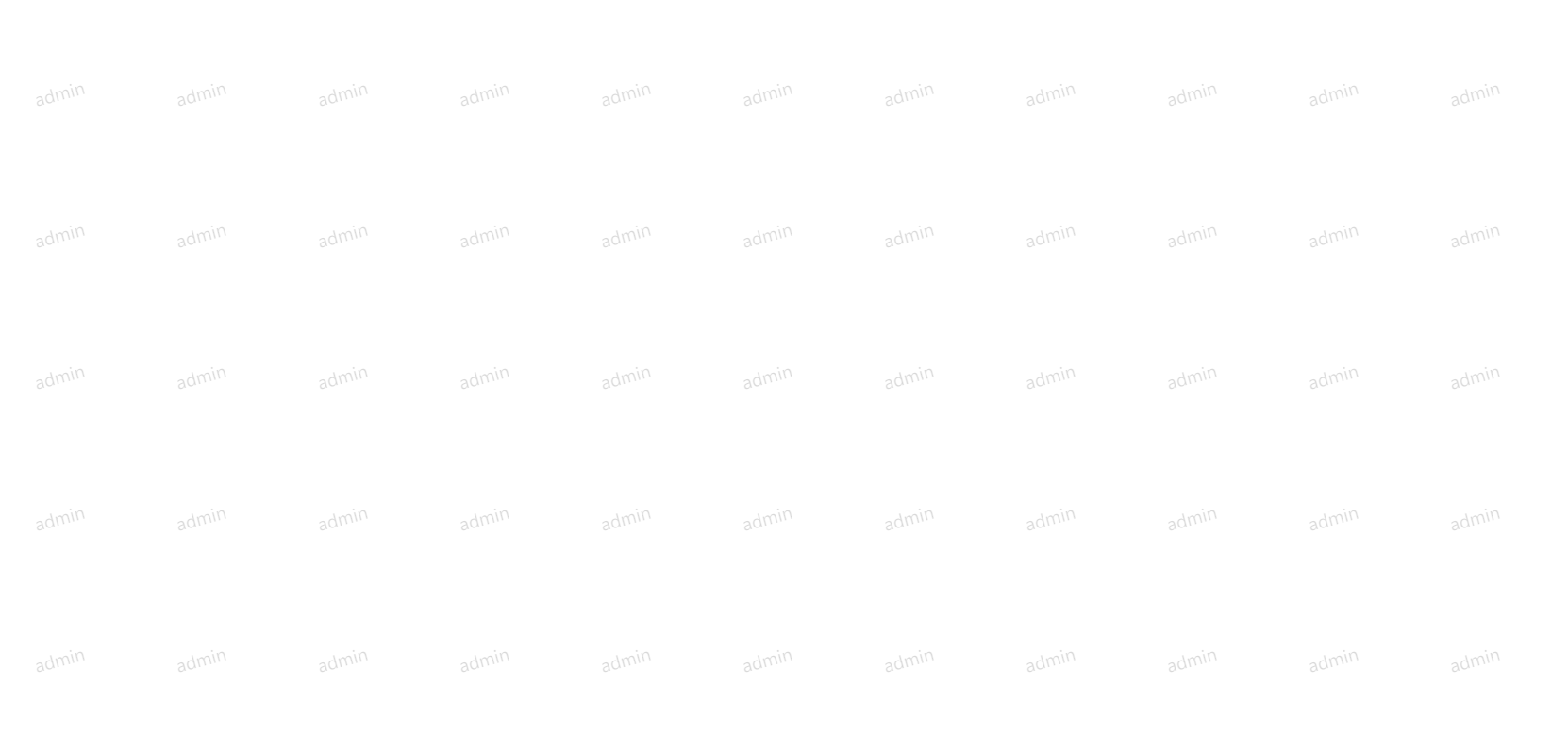
代码地址https://github.com/saucxs/watermark-dom.git
无论哪种方法,都可以帮助您保护您的Vue网站内容并确保其安全性。希望这篇文章能够对您有所帮助!